ESP8266 Part 3
UDP client
The goal with this article is to show how to set up the ESP8266 as a UDP client. This example can be used to transmit data at a constant rate being acquired by the ESP8266 to a remote UDP server. Have in mind that you might lose data during transmission. If your application cannot afford to lose packets or data, you should use a different approach (for example a TCP connection).
ESP 8266 UDP Client
We start by including the code from Part 2 in order to have the ESP8266 set up as an Access Point.
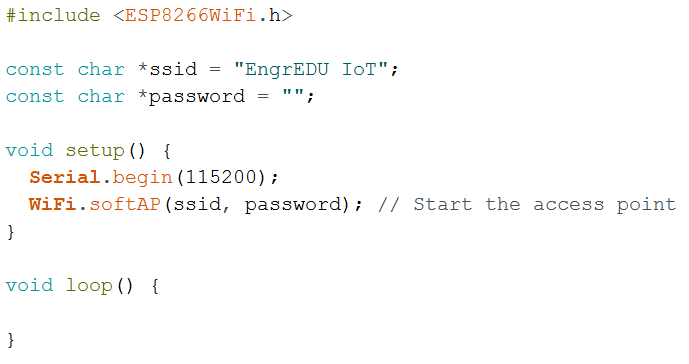
After that we include the WiFiUdp library to be able to create an UDP socket, and as in a normal UDP connection you need to provide the IP and port of the remote server that you would like to send the data to.
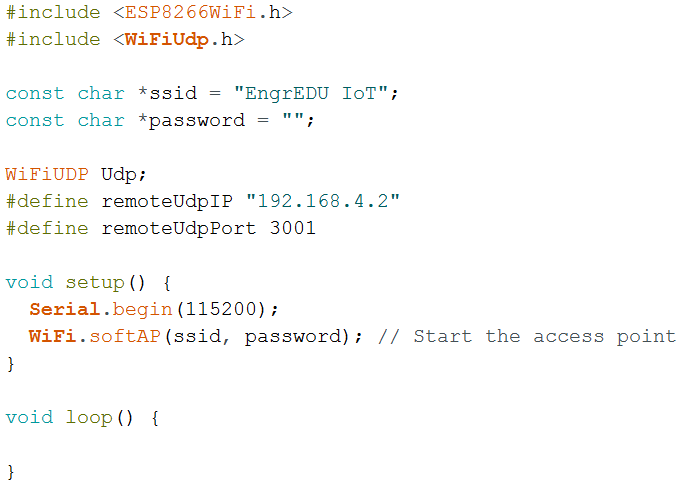
The rest of the code is fairly straight forward. Because you don't need to create a connection, you can simple send the data through the socket whenever you desire. For this particular example, we are creating a counter and sending that value to the server every 1 second. The counter gets converted to a string (using sprintf) and sent over the network using the write method of the socket. Make sure to have the write method between the beginPacket and endPacket methods.
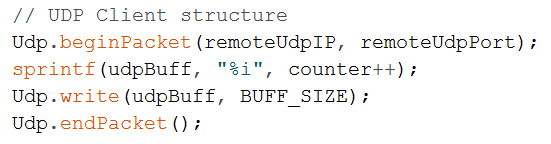
The full ESP8266 UDP client code is below.
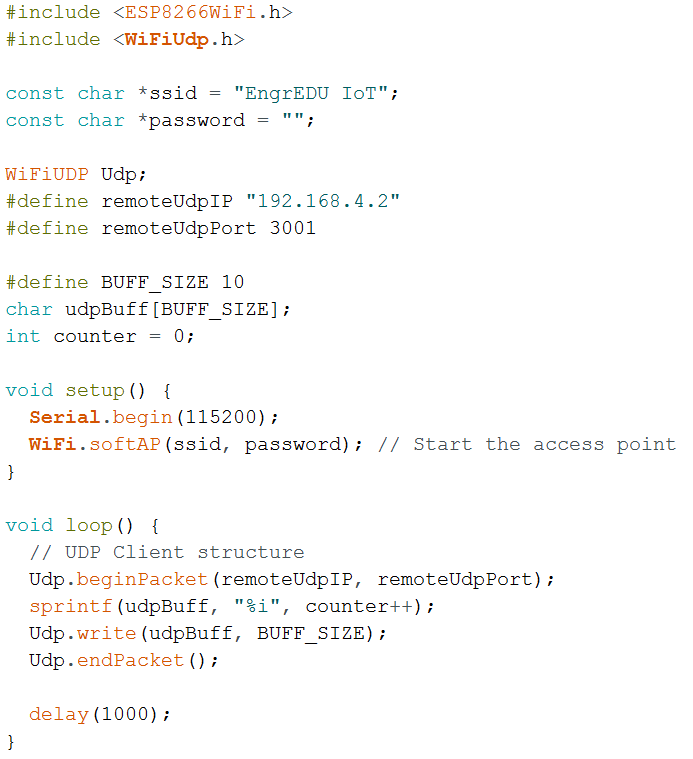
UDP Server (in Python)
Writing python code, specially code for network communications (UDP and TCP sockets) is outside of the scope of this article. It can be complex to develop a UDP server that handles a variety of different protocols and is robust to protocols or connections issues. However, below you can find a simple UDP server that you can use to test with the ESP8266 UDP client. Have in mind that you should change this code in order to be more tolerant to protocol or connection issues.
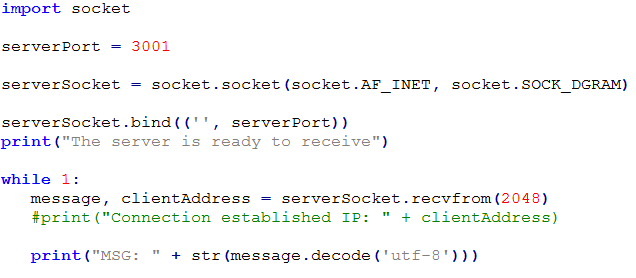
A couple of things to have in mind:
- Make sure that your computer is connected to the same WiFi network that you created with the ESP8266 (in this example EngrEDU IoT).
- Check the IP address that was assigned to the device that is being used as the server (computer or raspberry pi). In this example is "192.168.4.2"
- Use that server IP address in your remoteUdpIP variable(in your ESP8266 code).
- Use the same serverPort number (python code) as the number under remoteUdpPort (in your ESP8266 code) - in this case 3001.
- On windows computers you might need to configure your firewall to allow UDP communications to happen under that UDP port.
This should be sufficient to see some action happening. Now all you need to do is to focus on the protocol that you want to use for your application. Instead of sending the counter value, you can send whatever data you would like to.
Note: On AVR based boards, outgoing UDP packets are limited to 72 bytes in size currently. For non-AVR boards the limit is 1446 bytes [Reference].
Part 4 will go over on how to set up a TCP client on the ESP8266 side and transmit data to a TCP server on your computer.