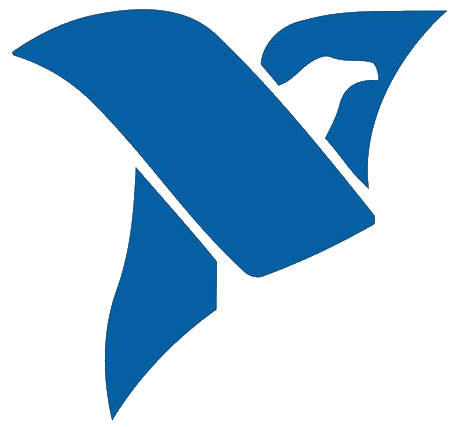
NI DAQs Intro
For this article I am using the NI USB 6001 (screw terminal) DAQ (Datasheet Link). If you are using any other NI USB model, the steps should be pretty much similar to the ones described here.
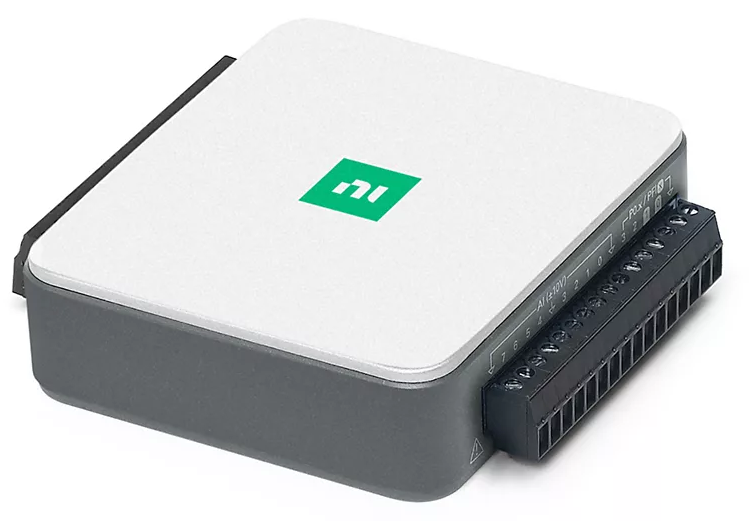
NI-DAQmx Drivers
If you have these drivers installed already, you can skip this section.
To run NI-MAX and use Python to interact with the DAQ, we need to install the NI-DAQmx drivers first (Link).
NI-DAQmx is an NI instrument driver that controls every aspect of your DAQ system, including signal conditioning, from configuration to programming in LabVIEW to low-level OS and device control.
The nidaqmx package contains an API (Application Programming Interface) for interacting with the NI-DAQmx driver. The package is implemented in Python. The package is implemented as a complex, highly object-oriented wrapper around the NI-DAQmx C API using the ctypes Python library.
NI-MAX
Before writing any code, the first thing that I like to do is to run NI-MAX to configure the DAQ, change the name of the DAQ (if necessary), and run some predefined tasks to see if the drivers for the board are installed properly.
Note: NI MAX is only supported on Windows and MacOS, not Linux, and cannot be downloaded by itself. It is included with all NI drivers (NI-VISA, NI-DAQmx etc.) and NI System Configuration.
Start NI-MAX and the window below will show up. You can interact with the active DAQ connected to your computer by expanding the "Devices and Interfaces" menu, and selecting the respective DAQ model that is connected to your computer. You can change the name of the DAQ to something more meaningful to your project, and you can run analog and/or digital tasks to test if the boards is running properly.
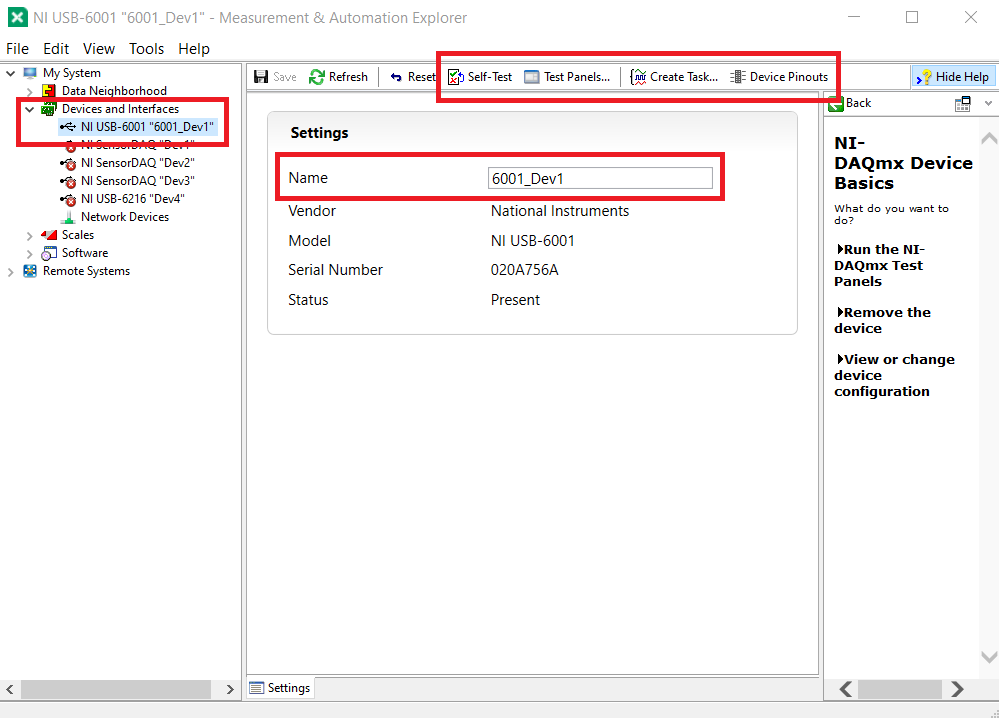
I use NI-MAX Test Panels functionality to get an idea on how the board works, and use that knowledge to help while interacting with the DAQ in Python.
Python Example
You can use any IDE of your choice, just make sure that you know how to install the necessary python packages for the IDE of your choice. I will be using PyCharm, and for this particular example make sure that you install nidaqmx package for python.
Let's write our first code to interact with the DAQ and check if everything is install properly. I will be using this code as a reference (Link).
import nidaqmx
from nidaqmx.system import System
def get_DAQ_Info():
# Get local system object
local_system = System.local()
# Get version info
driver_version = local_system.driver_version
print("DAQmx {0}.{1}.{2}".format(driver_version.major_version, driver_version.minor_version,
driver_version.update_version))
# Get Device Names
for device in local_system.devices:
print("Device Name: {0}, Product Category: {1}, Product Type: {2}".format(
device.name, device.product_category, device.product_type
))
if __name__ == "__main__":
get_DAQ_Info()
If the nidaqmx package is installed properly you will get the output below.
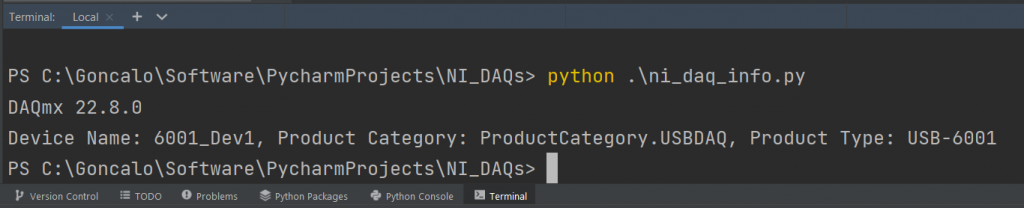
Python Files
References
- [Ref 1] “NI-DAQmx Python Tutorial”, nspyre-org Website [Articles]
- [Ref 2] “Using a NI DAQ Device with Python and NI DAQmx”, NI Website [Article]
- [Ref 3] “NI-DAQmx Key Concepts”, documentation.HELP! Website
- [Ref 4] “nidaqmx_examples”, GitHub